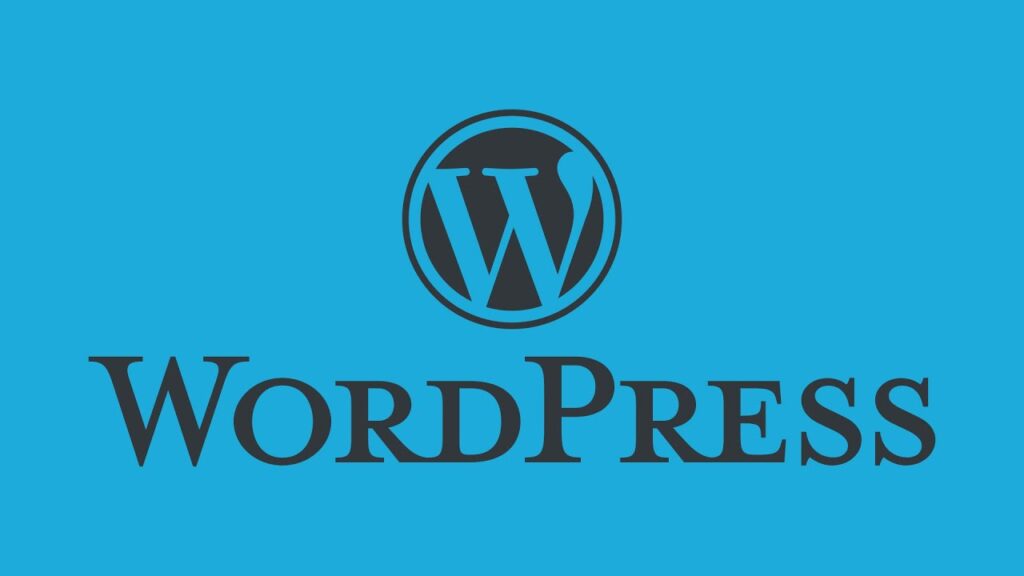
WordPress development has evolved significantly, and modern tooling plays a crucial role in creating efficient and maintainable projects. wp-scripts
is a powerful package that simplifies the development process, providing a standardized and streamlined way to build themes and plugins utilizing modern JavaScript, CSS, and other front-end technologies. This comprehensive guide will walk you through how to leverage wp-scripts
for your next WordPress project.
What is wp-scripts
?
wp-scripts
is a zero-configuration build tool built specifically for WordPress development. It’s included with the @wordpress/scripts
npm package and aims to provide a consistent and reliable development experience by handling common tasks like:
- Transpilation: Compiling modern JavaScript (ESNext) with Babel to ensure compatibility with older browsers.
- Bundling: Combining JavaScript modules into optimized bundles using Webpack.
- Linting: Enforcing code style and identifying potential errors using ESLint.
- Formatting: Automatically formatting code using Prettier to ensure consistency.
- CSS Processing: Compiling and processing CSS with PostCSS, allowing for features like Autoprefixer.
- BrowserSync: Providing hot-reloading and browser synchronization for a smooth development workflow.
Why Use wp-scripts
?
- Zero Configuration: Get started quickly without spending time configuring complex build tools.
wp-scripts
provides sensible defaults for common WordPress development scenarios. - Consistency: Enforces a consistent development workflow across different projects, making it easier to collaborate and maintain code.
- Modern Tooling: Leverages industry-standard tools like Webpack, Babel, ESLint, and Prettier.
- Easy Updates: Keep your development tooling up-to-date easily through npm.
- WordPress Standards: Aligned with WordPress coding standards and best practices.
Setting Up a Project with wp-scripts
- Project Initialization: Create a new directory for your project and navigate to it in your terminal.
mkdir my-wp-project cd my-wp-project
Package Initialization: Initialize a new package.json
file using npm.
npm init -y
Install @wordpress/scripts
: Install the @wordpress/scripts
package as a development dependency.
npm install @wordpress/scripts --save-dev
Configure package.json
: Add scripts to your package.json
file to run various wp-scripts
commands. The most common scripts are start
, build
, and lint
.
{ "name": "my-wp-project", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "start": "wp-scripts start", "build": "wp-scripts build", "lint:js": "wp-scripts lint-js", "lint:css": "wp-scripts lint-style", "packages-update": "wp-scripts packages-update" }, "keywords": [], "author": "", "license": "ISC", "devDependencies": { "@wordpress/scripts": "^26.3.0" } }
-
start
: Starts the development server and watches for file changes.build
: Creates production-ready bundles of your code.lint:js
: Lints JavaScript files using ESLint.lint:css
: Lints CSS files using Stylelint.packages-update
: Updates all WordPress packages to the latest version.
Project Structure
While wp-scripts
doesn’t enforce a strict project structure, a recommended approach is:
my-wp-project/ ├── src/ # Source files │ ├── index.js # Entry point for JavaScript │ ├── style.scss # Main stylesheet │ └── blocks/ # Directory for custom blocks (if applicable) ├── dist/ # Compiled output (automatically generated) ├── package.json ├── package-lock.json ├── node_modules/ # Dependencies (automatically generated) └── .eslintrc.js # ESLint configuration (optional)
Basic Usage: Styling
Let’s create a simple stylesheet.
- Create
src/style.scss
: Create a new file atsrc/style.scss
and add some basic CSS.
body { font-family: sans-serif; background-color: #f0f0f0; padding: 20px; } h1 { color: #333; }
Enqueue the Stylesheet: In your WordPress theme’s functions.php
file (or plugin main file), enqueue the compiled CSS file.
<?php function my_wp_project_enqueue_assets() { wp_enqueue_style( 'my-wp-project-style', get_stylesheet_directory_uri() . '/dist/style.css', // Adjust to your theme directory if necessary array(), filemtime( get_stylesheet_directory() . '/dist/style.css' ) // Cache busting ); } add_action( 'wp_enqueue_scripts', 'my_wp_project_enqueue_assets' );
- Run
npm start
ornpm run build
: Executenpm start
in your terminal to start the development server with hot-reloading.wp-scripts
will automatically compile yourscss
file todist/style.css
. Alternatively, usenpm run build
to create a production-ready version.
Basic Usage: JavaScript
- Create
src/index.js
: Create a new file atsrc/index.js
and add some JavaScript code.
console.log('Hello from wp-scripts!'); document.addEventListener('DOMContentLoaded', function() { const heading = document.querySelector('h1'); if (heading) { heading.textContent = 'Hello World!'; } });
2. Enqueue the Script: Enqueue the compiled JavaScript file in your WordPress theme’s functions.php
file (or plugin main file).
<?php function my_wp_project_enqueue_assets() { wp_enqueue_script( 'my-wp-project-script', get_stylesheet_directory_uri() . '/dist/index.js', // Adjust to your theme directory if necessary array(), filemtime( get_stylesheet_directory() . '/dist/index.js' ), // Cache busting true // Place script in the footer ); } add_action( 'wp_enqueue_scripts', 'my_wp_project_enqueue_assets' );
- Run
npm start
ornpm run build
: Just like with the styling, executenpm start
to start the development server ornpm run build
to create a production-ready bundle.wp-scripts
will compile your JavaScript code, bundle it, and place the output indist/index.js
.
Working with Blocks
wp-scripts
is particularly well-suited for developing custom Gutenberg blocks. Here’s how to set up a basic block:
- Create a Block Directory: Inside your
src
directory, create a new directory for your blocks, e.g.,src/blocks/
. - Create Block Files: Inside the
src/blocks/
directory, create two files for your block:block.js
(for JavaScript) andblock.scss
(for CSS).src/blocks/block.js
:
import { registerBlockType } from '@wordpress/blocks'; import './block.scss'; registerBlockType( 'my-wp-project/my-custom-block', { title: 'My Custom Block', icon: 'smiley', category: 'common', edit: () => { return <p>Hello from the editor!</p>; }, save: () => { return <p>Hello from the frontend!</p>; }, } );
2. src/blocks/block.scss
:
.wp-block-my-wp-project-my-custom-block { background-color: lightblue; padding: 10px; }
3. Import Block in index.js
: Import your block into src/index.js
to register it.
import './blocks/block.js'; // Import your block console.log('Hello from wp-scripts!'); document.addEventListener('DOMContentLoaded', function() { const heading = document.querySelector('h1'); if (heading) { heading.textContent = 'Hello World!'; } });
- Run
npm start
ornpm run build
: Runningnpm start
ornpm run build
will now automatically bundle your block code, making it available in the Gutenberg editor.
Advanced Configuration (Optional)
While wp-scripts
aims for zero configuration, you can customize its behavior by creating configuration files in your project root:
- .eslintrc.js: Customize ESLint rules.
- .prettierrc.js: Customize Prettier formatting rules.
- webpack.config.js: Customize the underlying Webpack configuration. This provides the most flexibility but requires a deeper understanding of Webpack. Use with caution as incorrect modifications can break the build process. You can create this file by running
wp-scripts eject
, this will give you the full webpack configuration.
Example: Customizing ESLint
- Create
.eslintrc.js
: Create a file named.eslintrc.js
in your project root. - Add Configuration: Add your desired ESLint rules. For example, to allow
console.log
statements:
module.exports = { "extends": "@wordpress/eslint-plugin", "rules": { "no-console": "off" // Disable the no-console rule } };
Common Issues and Troubleshooting
- “Command not found: wp-scripts”: Ensure you’ve installed
@wordpress/scripts
as a development dependency (npm install @wordpress/scripts --save-dev
) and that yournode_modules
directory is present. - “Module not found…”: Verify that the modules you’re importing are installed (
npm install module-name
). - CSS Changes Not Reflecting: Make sure your CSS is properly enqueued in your theme’s
functions.php
file and that the file path is correct. Also, check your browser’s cache. - JavaScript Errors: Inspect your browser’s developer console for JavaScript errors.
- “TypeError: Cannot read properties of undefined (reading ‘context’)”: This often happens when calling
wp-scripts
commands outside of the project root directory. Ensure you’re in the directory containingpackage.json
.
Conclusion
wp-scripts
offers a streamlined and efficient approach to modern WordPress development. By leveraging its zero-configuration design and powerful tooling, you can focus on building innovative and high-quality themes and plugins without the complexities of managing intricate build processes. By following this guide, you can start building your next WordPress project with confidence using wp-scripts
. Remember to consult the official @wordpress/scripts
documentation for the most up-to-date information and advanced configuration options.