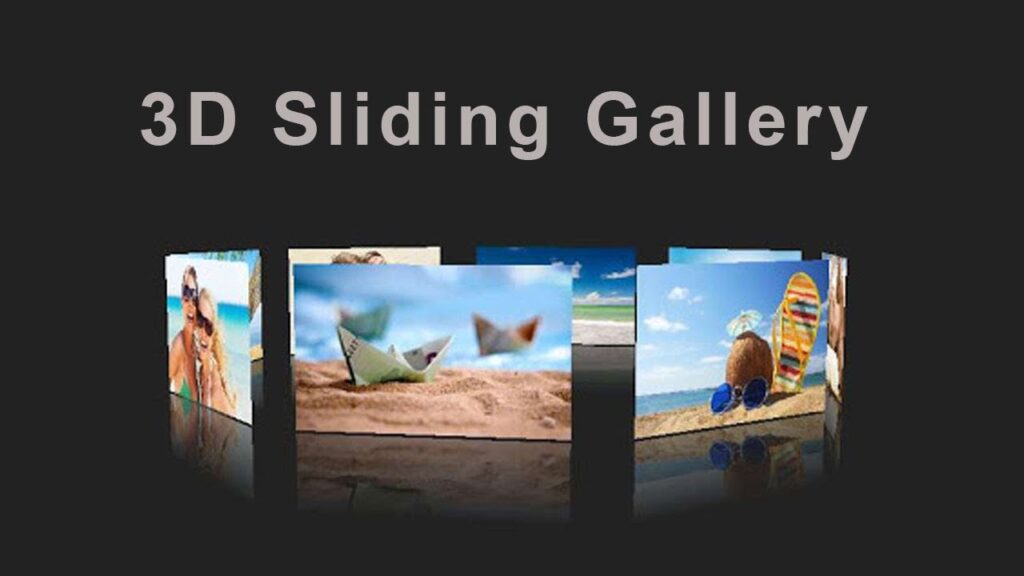
Creating a 3D image slider can be an exciting way to showcase images with a dynamic effect. Below is an example of how to implement a simple 3D image slider using HTML, CSS, and JavaScript.
HTML Structure (index.html)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="style.css"> <title>3D Image Slider</title> </head> <body> <div class="slider"> <div class="slides"> <div class="slide active"><img src="image1.jpg" alt="Image 1"></div> <div class="slide"><img src="image2.jpg" alt="Image 2"></div> <div class="slide"><img src="image3.jpg" alt="Image 3"></div> </div> <button class="prev" onclick="prevSlide()">❮</button> <button class="next" onclick="nextSlide()">❯</button> </div> <script src="script.js"></script> </body> </html>
CSS Styles (style.css)
body { margin: 0; overflow: hidden; background-color: #333; } .slider { position: relative; width: 80%; max-width: 600px; perspective: 1000px; margin: auto; height: 400px; } .slides { display: flex; transition: transform 0.6s; transform-style: preserve-3d; } .slide { min-width: 100%; transition: transform 1s; position: relative; backface-visibility: hidden; } .slide img { width: 100%; height: 100%; border-radius: 10px; } .active { transform: rotateY(0); } .slide:not(.active) { transform: rotateY(90deg); } .button { position: absolute; top: 50%; transform: translateY(-50%); background: rgba(255, 255, 255, 0.7); border: none; cursor: pointer; font-size: 30px; border-radius: 5px; } .prev { left: 10px; } .next { right: 10px; }
JavaScript Logic (script.js)
let currentIndex = 0; const slides = document.querySelectorAll('.slide'); const totalSlides = slides.length; function showSlide(index) { slides.forEach((slide, i) => { slide.classList.remove('active'); if (i === index) { slide.classList.add('active'); } }); } function nextSlide() { currentIndex = (currentIndex + 1) % totalSlides; // loop back to first slide showSlide(currentIndex); } function prevSlide() { currentIndex = (currentIndex - 1 + totalSlides) % totalSlides; // loop back to last slide showSlide(currentIndex); } // Automatically change slide every 5 seconds setInterval(nextSlide, 5000);
Explanation
- HTML Structure:
- The slider contains a list of images wrapped in a container with class
.slides
. Each image is in a slide div with class.slide
. - There are “previous” (❮) and “next” (❯) buttons that allow users to navigate through the slides.
- The slider contains a list of images wrapped in a container with class
- CSS Styles:
- We utilize
perspective
on the.slider
to give a 3D effect. - The
.slides
is a flex container that allows the slides to be placed side by side in a row. - Each slide has a transform property that sets its 3D rotation. The active slide is rotated to
0
, while the others are rotated90deg
to make them invisible.backface-visibility: hidden;
ensures that the backside of the images is not visible.
- We utilize
- JavaScript Logic:
- The
showSlide
function updates the active slide based on the current index. - The
nextSlide
andprevSlide
functions increment or decrement thecurrentIndex
and callshowSlide
to update the displayed slide. - An interval is set to automatically transition to the next slide every 5 seconds.
- The
Usage
- Replace
"image1.jpg"
,"image2.jpg"
, and"image3.jpg"
in the HTML file with actual image paths that you wish to showcase. - Open your
index.html
file in a web browser to view the 3D slider in action.
This implementation provides a basic foundation. You can enhance it further by adding more styling, controls, animations, or integration with keyboard events for accessibility.