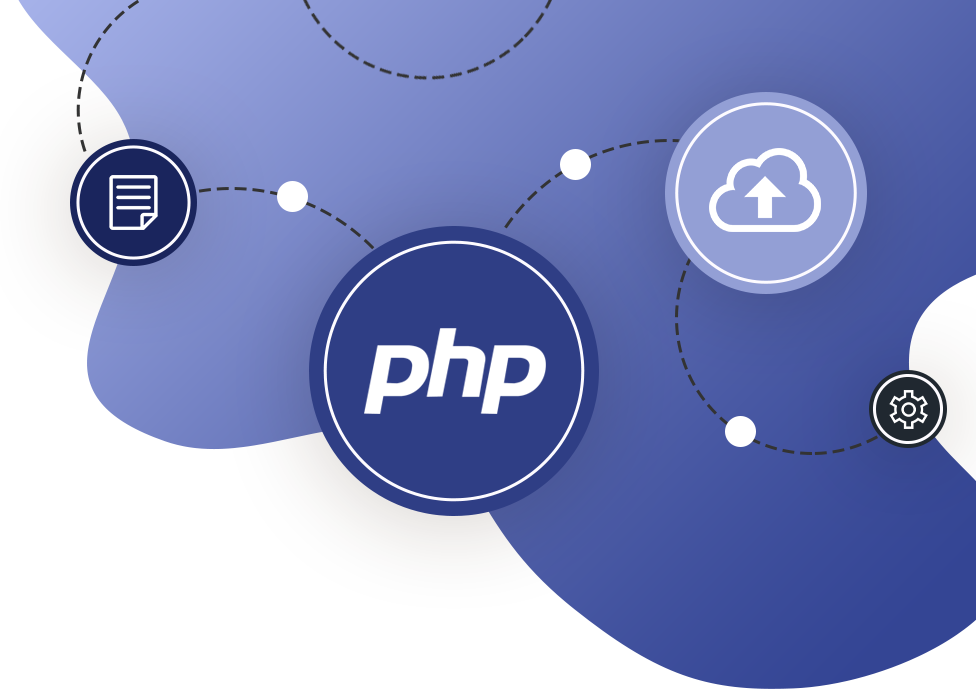
Operator Precedence
When you have a complex expression in PHP involving multiple operators, the order in which these operators are evaluated becomes crucial. This is determined by operator precedence. Operators with higher precedence are evaluated before operators with lower precedence. For example, multiplication (*) and division (/) have higher precedence than addition (+) and subtraction (-).
$result = 2 + 3 * 4; // Evaluates to 14 because multiplication is done first.
You can use parentheses ()
to explicitly control the order of evaluation. Expressions within parentheses are always evaluated first.
$result = (2 + 3) * 4; // Evaluates to 20 because the addition is done first, due to the parentheses.
It’s good practice to use parentheses even when you know the default precedence, as it greatly improves readability and reduces the chance of errors. Refer to the PHP documentation for a complete table of operator precedence.
Operator Associativity
When you have multiple operators of the same precedence in an expression, operator associativity comes into play. Associativity determines whether the operators are grouped from left to right or from right to left.
- Left-associative: Operators are grouped from left to right. Most binary operators are left-associative (e.g., +, -, *, /).
$result = 10 - 5 - 2; // Evaluates as (10 - 5) - 2, resulting in 3.
Right-associative: Operators are grouped from right to left. Assignment operators (=, +=, -=, etc.) are right-associative.
$a = $b = 5; // Evaluates as $a = ($b = 5), so both $a and $b become 5.
Common Types of PHP Operators
Beyond the basic arithmetic and logical operators, PHP offers a wide range of operators:
- Assignment Operators: Used to assign values to variables. Examples:
=
,+=
,-=
,*=
,/=
,%=
,.=
. The.=
operator is used for string concatenation. php
$name = "John"; $name .= " Doe"; // $name is now "John Doe"
Comparison Operators: Used to compare two values. Examples: ==
(equal), !=
(not equal), ===
(identical – equal and of the same type), !==
(not identical), <
(less than), >
(greater than), <=
(less than or equal to), >=
(greater than or equal to), <>
(not equal – same as !=
).
if ($age >= 18) { echo "You are an adult."; }
Incrementing/Decrementing Operators: Used to increase or decrease the value of a variable by one. Examples: ++$x
(pre-increment), $x++
(post-increment), --$x
(pre-decrement), $x--
(post-decrement). The pre-increment/decrement operators modify the value before returning it, while the post-increment/decrement operators modify the value after returning it.
$x = 5; echo ++$x; // Output: 6. $x is now 6. $y = 5; echo $y++; // Output: 5. $y is now 6.
Logical Operators: Used to combine or negate boolean expressions. Examples: &&
(logical AND), ||
(logical OR), !
(logical NOT), and
(logical AND – lower precedence than &&
), or
(logical OR – lower precedence than ||
), xor
(logical XOR – exclusive OR).
if (($age >= 18) && ($country == "USA")) { echo "You are an adult in the USA."; }
String Operators: PHP supports two string operators: .
(concatenation) and .=
(concatenation assignment).
$firstName = "John"; $lastName = "Doe"; $fullName = $firstName . " " . $lastName; // $fullName is "John Doe"
Array Operators: Used to compare arrays. Examples: +
(union), ==
(equality), ===
(identity), !=
(inequality), <>
(inequality), !==
(non-identity). The union operator appends the right-hand array to the left-hand array; keys that exist in both arrays will keep the value from the left-hand array.
$a = array("a" => "red", "b" => "green"); $b = array("c" => "blue", "d" => "yellow"); $c = $a + $b; // $c contains a union of $a and $b.
- Bitwise Operators: Allow you to manipulate individual bits within an integer. Examples:
&
(AND),|
(OR),^
(XOR),~
(NOT),<<
(left shift),>>
(right shift). These are less commonly used in typical web development but are important for certain types of low-level operations. - Error Control Operators: The
@
operator. When prepended to an expression, any error messages that might be generated by that expression will be suppressed. Use this operator sparingly as it can mask underlying problems. It’s generally better to handle errors explicitly usingtry...catch
blocks or custom error handlers.
Understanding these operators, their precedence, and their associativity is fundamental to writing correct and efficient PHP code. Always refer to the official PHP documentation for the most accurate and up-to-date information.