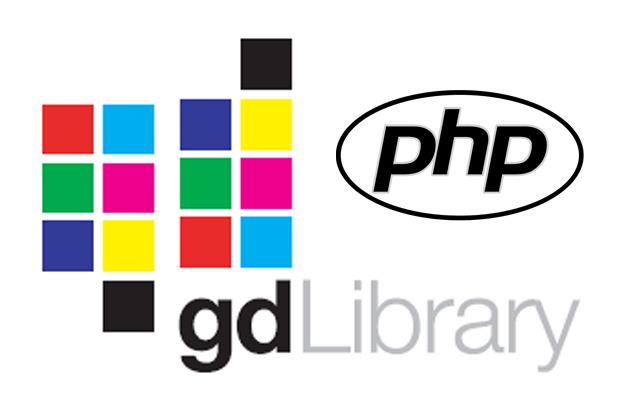
Resizing and changing the format of an image using PHP’s GD library involves loading the image, creating a new true color image with the desired dimensions, and then saving it in the desired format. Let’s walk through a detailed example.
<?php class ImageProcessor { private $sourcePath; private $destinationPath; private $newWidth; private $newHeight; public function __construct($sourcePath, $destinationPath, $newWidth, $newHeight) { $this->sourcePath = $sourcePath; $this->destinationPath = $destinationPath; $this->newWidth = $newWidth; $this->newHeight = $newHeight; } // Load an image from a file private function loadImage($filePath) { $imageInfo = getimagesize($filePath); switch ($imageInfo[2]) { case IMAGETYPE_JPEG: return imagecreatefromjpeg($filePath); case IMAGETYPE_PNG: return imagecreatefrompng($filePath); case IMAGETYPE_GIF: return imagecreatefromgif($filePath); default: throw new Exception("Unsupported image type."); } } // Resize and change image format public function resizeAndChangeFormat($newFormat = 'jpeg') { // Load the original image $sourceImage = $this->loadImage($this->sourcePath); // Create a new true color image with the specified dimensions $resizedImage = imagecreatetruecolor($this->newWidth, $this->newHeight); // Get original dimensions $originalWidth = imagesx($sourceImage); $originalHeight = imagesy($sourceImage); // Resize the original image into the new image imagecopyresampled($resizedImage, $sourceImage, 0, 0, 0, 0, $this->newWidth, $this->newHeight, $originalWidth, $originalHeight); // Output the image in the desired format switch (strtolower($newFormat)) { case 'jpeg': case 'jpg': imagejpeg($resizedImage, $this->destinationPath); break; case 'png': imagepng($resizedImage, $this->destinationPath); break; case 'gif': imagegif($resizedImage, $this->destinationPath); break; default: throw new Exception("Unsupported output format."); } // Free up memory imagedestroy($sourceImage); imagedestroy($resizedImage); } } // Usage example try { $source = '/path/to/source/image.jpg'; // Path to source image $destination = '/path/to/destination/image.png'; // Path to save the new image $width = 200; // New width $height = 100; // New height $format = 'png'; // The desired output format $processor = new ImageProcessor($source, $destination, $width, $height); $processor->resizeAndChangeFormat($format); echo "Image resized and format changed successfully!"; } catch (Exception $e) { echo "Error: " . $e->getMessage(); }
Explanation:
- Initialization:
- The
ImageProcessor
class is initialized with source and destination paths, and the desired width and height for the new image.
- The
- Loading the Image:
- The
loadImage()
method checks the image type usinggetimagesize()
and loads it with the appropriate GD function:imagecreatefromjpeg()
,imagecreatefrompng()
, orimagecreatefromgif()
. - If the image type is unsupported, an exception is thrown.
- The
- Resizing the Image:
- A new true color image is created with
imagecreatetruecolor()
for the target dimensions. imagesx()
andimagesy()
functions retrieve the original dimensions of the source image.imagecopyresampled()
handles the actual resizing, maintaining quality by using a resampling algorithm.
- A new true color image is created with
- Changing the Format:
- The resized image is saved in the specified format using
imagejpeg()
,imagepng()
, orimagegif()
. - Again, an unsupported format triggers an exception.
- The resized image is saved in the specified format using
- Memory Management:
imagedestroy()
is called for both the original and resized images to free up resources.
- Error Handling:
- The entire process is wrapped in a try-catch block to handle exceptions gracefully.
Considerations:
- Ensure the GD library is installed and enabled in your PHP environment.
- Adjust the destination path and format according to your requirements.
- Support additional image formats as necessary by extending the functionalities in
loadImage()
andresizeAndChangeFormat()
.