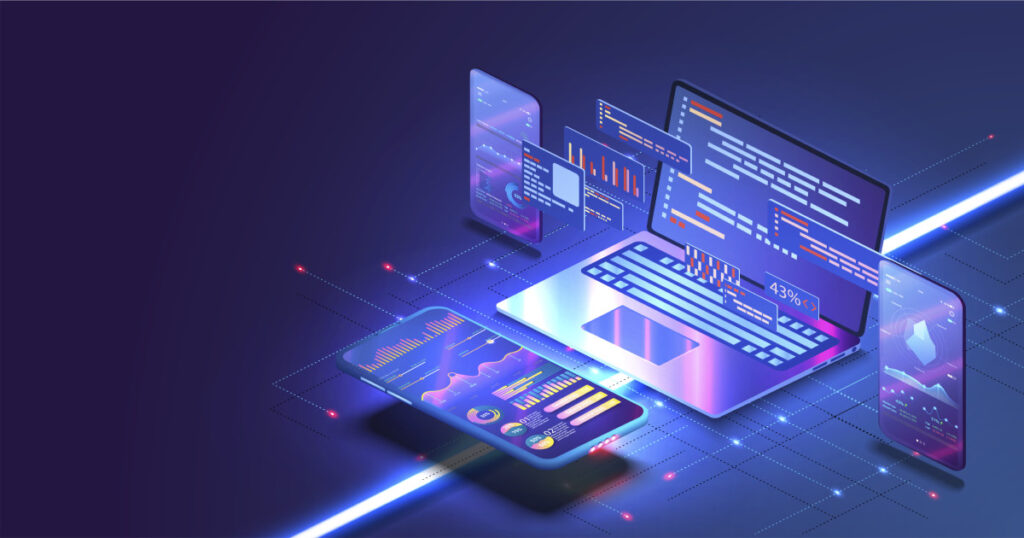
Below is a simple yet effective script that enlarges images when they are clicked on a website. This example uses HTML, CSS, and JavaScript to create a lightbox effect, allowing users to click on an image to view it in a larger size without leaving the page.
Step-by-Step Guide
Step 1: HTML Structure
Create an HTML file (e.g., index.html
) with the following structure:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Image Gallery</title> <link rel="stylesheet" href="styles.css"> </head> <body> <h1>Image Gallery</h1> <div class="gallery"> <img src="image1.jpg" alt="Image 1" class="thumbnail"> <img src="image2.jpg" alt="Image 2" class="thumbnail"> <img src="image3.jpg" alt="Image 3" class="thumbnail"> <!-- Add more images as needed --> </div> <div id="lightbox" class="lightbox"> <span class="close">×</span> <img class="lightbox-content" id="lightbox-img" src=""> </div> <script src="script.js"></script> </body> </html>
Step 2: CSS Styling
Create a CSS file (e.g., styles.css
) to style the gallery and the lightbox:
body { font-family: Arial, sans-serif; } .gallery { display: flex; flex-wrap: wrap; gap: 10px; } .thumbnail { width: 150px; height: auto; cursor: pointer; transition: transform 0.2s; } .thumbnail:hover { transform: scale(1.05); } .lightbox { display: none; position: fixed; z-index: 1000; left: 0; top: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.8); justify-content: center; align-items: center; } .lightbox-content { max-width: 90%; max-height: 90%; } .close { position: absolute; top: 20px; right: 30px; color: white; font-size: 40px; cursor: pointer; }
Step 3: JavaScript Functionality
Create a JavaScript file (e.g., script.js
) that will handle the lightbox functionality:
// Get the lightbox element const lightbox = document.getElementById('lightbox'); const lightboxImg = document.getElementById('lightbox-img'); const closeBtn = document.querySelector('.close'); // Add click event to each thumbnail document.querySelectorAll('.thumbnail').forEach(image => { image.addEventListener('click', () => { lightbox.style.display = 'flex'; // Show lightbox lightboxImg.src = image.src; // Set the src of the lightbox image }); }); // Adding event listener to close button closeBtn.addEventListener('click', () => { lightbox.style.display = 'none'; // Hide lightbox }); // Hide the lightbox when clicking outside the image lightbox.addEventListener('click', (event) => { if (event.target === lightbox) { lightbox.style.display = 'none'; // Hide lightbox if clicked outside the image } });
How It Works
- HTML Structure:
- A gallery of thumbnails is set up using
<div>
and<img>
tags. - A hidden lightbox (
<div>
with IDlightbox
) is created to display the enlarged image.
- A gallery of thumbnails is set up using
- CSS Styles:
- Basic styles are applied to format the layout of the gallery.
- The lightbox is styled to cover the entire screen with a semi-transparent background, making it overlay other content.
- The close button is styled for easy visibility.
- JavaScript Functionality:
- When a thumbnail is clicked, an event listener triggers, displaying the lightbox and setting the source of the enlarged image to the clicked thumbnail’s source.
- The close button and clicking outside the image hide the lightbox.
How to Use
- Replace
image1.jpg
,image2.jpg
, etc., with the paths to your actual images. - Open
index.html
in a web browser. - Click on any thumbnail to see the enlarged image in the lightbox. Click the close button or outside the image to exit the lightbox.
This script gives you a simple yet elegant way to showcase images on your website, enhancing user experience while navigating through your gallery! Feel free to modify styles and functionality according to your project needs.